在《【Linux】利用C语言文件流复制单一文件》(http://www.linuxdiyf.com/linux/17721.html)讲述了如何用C语言拷贝文件,但是这只能拷贝单一文件。如果你要用LinuxC拷贝整个文件夹,同样要像《【Java】利用文件输入输出流完成把一个文件夹内的所有文件拷贝的另一的文件夹的操作》(附1)一样,先用《【Linux】遍历某一目录,判断文件与文件夹,main参数》(http://www.linuxdiyf.com/linux/17718.html)的方法遍历整个文件目录,之后再一个一个实现文件拷贝。
具体代码如下:
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<dirent.h>//输出文件信息
#include<sys/stat.h>//判断是否目录
int is_dir(char* path){//判断是否是目录
struct stat st;
stat(path,&st);
if(S_ISDIR(st.st_mode)){
return 1;
}
else{
return 0;
}
}
/*字符串处理函数*/
int endwith(char* s,char c){//用于判断字符串结尾是否为“/”
if(s[strlen(s)-1]==c){
return 1;
}
else{
return 0;
}
}
char* str_contact(char* str1,char* str2){//字符串连接
char* result;
result=(char*)malloc(strlen(str1)+strlen(str2)+1);//str1的长度+str2的长度+\0;
if(!result){//如果内存动态分配失败
printf("字符串连接时,内存动态分配失败\n");
exit(1);
}
strcat(result,str1);
strcat(result,str2);//字符串拼接
return result;
}
/*复制函数*/
void copy_file(char* source_path,char *destination_path){//复制文件
char buffer[1024];
FILE *in,*out;//定义两个文件流,分别用于文件的读取和写入int len;
if((in=fopen(source_path,"r"))==NULL){//打开源文件的文件流
printf("源文件打开失败!\n");
exit(1);
}
if((out=fopen(destination_path,"w"))==NULL){//打开目标文件的文件流
printf("目标文件创建失败!\n");
exit(1);
}
int len;//len为fread读到的字节长
while((len=fread(buffer,1,1024,in))>0){//从源文件中读取数据并放到缓冲区中,第二个参数1也可以写成sizeof(char)
fwrite(buffer,1,len,out);//将缓冲区的数据写到目标文件中
}
fclose(out);
fclose(in);
}
void copy_folder(char* source_path,char *destination_path){//复制文件夹
if(!opendir(destination_path)){
if (mkdir(destination_path,0777))//如果不存在就用mkdir函数来创建
{
printf("创建文件夹失败!");
}
}
char *path;
path=(char*)malloc(512);//相当于其它语言的String path="",纯C环境下的字符串必须自己管理大小,这里为path直接申请512的位置的空间,用于目录的拼接
path=str_contact(path,source_path);//这三句,相当于path=source_path
struct dirent* filename;
DIR* dp=opendir(path);//用DIR指针指向这个文件夹
while(filename=readdir(dp)){//遍历DIR指针指向的文件夹,也就是文件数组。
memset(path,0,sizeof(path));
path=str_contact(path,source_path);
//如果source_path,destination_path以路径分隔符结尾,那么source_path/,destination_path/直接作路径即可
//否则要在source_path,destination_path后面补个路径分隔符再加文件名,谁知道你传递过来的参数是f:/a还是f:/a/啊?
char *file_source_path;
file_source_path=(char*)malloc(512);
if(!endwith(source_path,'/')){
file_source_path=str_contact(file_source_path,source_path);
file_source_path=str_contact(source_path,"/");
}
else{
file_source_path=str_contact(file_source_path,source_path);
}
char *file_destination_path;
file_destination_path=(char*)malloc(512);
if(!endwith(destination_path,'/')){
file_destination_path=str_contact(file_destination_path,destination_path);
file_destination_path=str_contact(destination_path,"/");
}
else{
file_destination_path=str_contact(file_destination_path,destination_path);
}
//取文件名与当前文件夹拼接成一个完整的路径
file_source_path=str_contact(file_source_path,filename->d_name);
file_destination_path=str_contact(file_destination_path,filename->d_name);
if(is_dir(file_source_path)){//如果是目录
if(!endwith(file_source_path,'.')){//同时并不以.结尾,因为Linux在所有文件夹都有一个.文件夹用于连接上一级目录,必须剔除,否则进行递归的话,后果无法相像
copy_folder(file_source_path,file_destination_path);//进行递归调用,相当于进入这个文件夹进行复制~
}
}
else{
copy_file(file_source_path,file_destination_path);//否则按照单一文件的复制方法进行复制。
printf("复制%s到%s成功!\n",file_source_path,file_destination_path);
}
}
}
/*主函数*/
int main(int argc,char *argv[]){
if(argv[1]==NULL||argv[1]==NULL){
printf("请输入两个文件夹路径,第一个为源,第二个为目的!\n");
exit(1);
}
char* source_path=argv[1];//取用户输入的第一个参数
char* destination_path=argv[2];//取用户输入的第二个参数
DIR* source=opendir(source_path);
DIR* destination=opendir(destination_path);
if(!source||!destination){
printf("你输入的一个参数或者第二个参数不是文件夹!\n");
}
copy_folder(source_path,destination_path);//进行文件夹的拷贝
return 0;
}
运行结果如下,设置一个有文件夹,有文件的文件夹A,计划将里面的内容,拷贝到原本空空是也的文件夹B中。
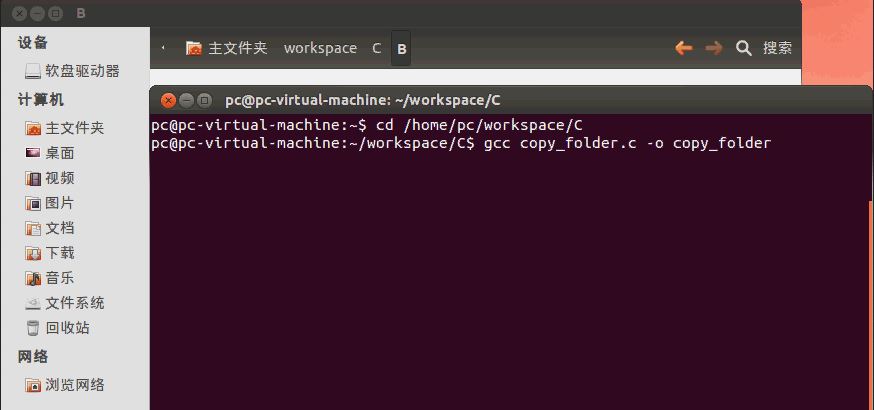
上述程序中,难点有以下几个:
1、由于此程序涉及多次文件路径的拼接,因此最大的难题就是C语言中对于字符串的处理。众所周知,C语言中是没有字符串的概念,仅有字符数组,与指向这个字符数组的首位置的指针的概念。用char *path;配合path=(char*)malloc(512);相当于搞出一个,指向长达512的空字符数组的指针,此时path你可以理解为字符串。这样使用的strcat函数,不停地给path进行拼接,就不会出现段错误的内存溢出错误。
接下去用了大量的代码用于源与目标文件路径与文件名的拼接,其实完成的功能很简单,如果是其它语言,可能string file_source_path;string filename->d_name;之后就一句file_source_path+filename->d_name;
反正大家明白什么回事就行了,不必细究,我也不过是把《【Linux】纯C环境下字符串的处理》(http://www.linuxdiyf.com/linux/17719.html)中方法搞过来。
2、还有一个问题,就是复制的时候,要注意排除当前文件夹中.与..这两个链接到上级目录与根目录的文件夹。在Linux似乎任何一个文件夹都有这个东东,一开始我写程序的时候,根本不知道,搞了一个复杂度超高还根本停不下来的递归迭代,这也是Linux与Windows不一样的地方。
3、其它地方也就没什么了,都是一些之前文件写过的东西。
附1:
