本例子虽小,但是融合的线程同步,线程回收和信号量的知识。
需要注意pthread_join()和pthread_exit()的用法和区别:
pthread_join一般是主线程来调用,用来等待子线程退出,因为是等待,所以是阻塞的,一般主线程会依次join所有它创建的子线程。
pthread_exit一般是子线程调用,用来结束当前线程。
子线程可以通过pthread_exit传递一个返回值,而主线程通过pthread_join获得该返回值,从而判断该子线程的退出是正常还是异常。
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
sem_t bin_sem;
int data;
void *thread_functionB(void *arg);
void *thread_functionA(void *arg);
int main(void)
{
int res;
pthread_t a_thread;
pthread_t b_thread;
void *thread_resultA;
void *thread_resultB;
if(sem_init(&bin_sem, 0, 0))
{
printf("sem init error!\n");
exit(EXIT_FAILURE);
}
res = pthread_create(&a_thread, NULL, thread_functionA, NULL);
if(res != 0)
{
perror("Thread creation failed");
exit(EXIT_FAILURE);
}
res = pthread_create(&b_thread, NULL, thread_functionB, NULL);
if(res != 0)
{
perror("thread creation failed!");
exit(EXIT_FAILURE);
}
//sem_wait(&bin_sem);
printf("input a number:\n");
scanf("%d",&data);
sem_post(&bin_sem);
if(pthread_join(a_thread, &thread_resultA)!=0)
{
perror("thread a join error!");
exit(EXIT_FAILURE);
}
if(pthread_join(b_thread, &thread_resultB)!=0)
{
perror("thread b join error!");
exit(EXIT_FAILURE);
}
printf("thread A has joined, it returned:%s\n", (char *)thread_resultA);
printf("thread B has joined, it returned:%s\n", (char *)thread_resultB);
printf("final data is:%d\n", data);
exit(EXIT_SUCCESS);
}
void *thread_functionA(void *arg)
{
sem_wait(&bin_sem);
data++;
printf("thread A ++\n");
sem_post(&bin_sem);
pthread_exit("thread A has exited");
}
void *thread_functionB(void *arg)
{
sem_wait(&bin_sem);
data++;
printf("thread B ++\n");
sem_post(&bin_sem);
pthread_exit("thread B has exited");
}
编译并运行结果正确:
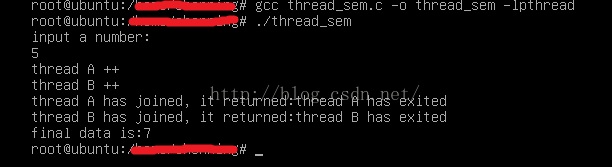