此示例是在Linux环境下(使用Linux系统编程线程相关函数)测试,文件说明如下:
ThreadBase.cpp, ThreadBase.h为线程基类
ThreadDerive.cpp, ThreadDerive.h为测试派生类
main.cpp为测试程序
ThreadBase.h内容如下:
#ifndef __THREADBASE_H__
#define __THREADBASE_H__
#include <pthread.h>
//线程基类
class ThreadBase
{
public:
ThreadBase(); //构造函数
virtual ~ThreadBase(); //虚析构函数
virtual int start(); //启动线程
virtual void routine(); //线程的运行函数,由派生类实现
virtual pthread_t self(); //获取当前线程的线程号
virtual int equal(pthread_t t); //比较线程号是否相等
virtual int detach(); //分离线程
virtual int join(pthread_t t); //连接线程
virtual int exit(); //线程退出
virtual int cancel(pthread_t t); //取消线程
virtual int destroy(); //销毁线程
private:
static void cleaner(void *pHandle); //线程清理函数
static void *work(void *pHandle); //线程回调函数
private:
pthread_attr_t t_attr; //线程属性
pthread_t tid; //线程号
};
#endif
ThreadBase.cpp内容如下:
#include <stdio.h>
#include "ThreadBase.h"
//构造函数,通过参数列表方式给线程号初始化为0
ThreadBase::ThreadBase():tid(0)
{
}
//虚析构函数
ThreadBase::~ThreadBase()
{
printf("~ThreadBase\n");
}
/****************************************************************
@功能:启动线程
@参数:无
@返回值: 成功:0,失败:-1
******************************************************************/
int ThreadBase::start()
{
int ret = 0;
size_t nsize = 1024*20; //20k
ret = pthread_attr_init(&t_attr); //初始化线程属性
if(ret != 0)
{
perror("pthread_attr_init");
return -1;
}
//设置线程的堆栈大小
ret = pthread_attr_setstacksize(&t_attr, nsize);
if(ret != 0)
{
perror("pthread_attr_getstacksize");
return -1;
}
//创建线程
return pthread_create(&tid, &t_attr, work, this);
}
/****************************************************************
@功能:线程的运行函数,此处为空,由派生类实现
@参数:无
@返回值: 无
******************************************************************/
void ThreadBase::routine()
{
}
/****************************************************************
@功能:获取当前线程的线程号
@参数:无
@返回值: 线程号
******************************************************************/
pthread_t ThreadBase::self()
{
if(!tid)
{
tid = pthread_self(); //获取线程号
}
return tid;
}
/****************************************************************
@功能:比较线程号是否相等
@参数:待比较的线程号
@返回值: 相等:0,不相等:-1
******************************************************************/
int ThreadBase::equal(pthread_t t)
{
int ret = 0;
ret = pthread_equal(tid, t);
return (ret)?0:-1;
}
/****************************************************************
@功能:分离线程,待线程结束时让系统自动回收其资源
@参数:无
@返回值: 成功:0,失败:-1
******************************************************************/
int ThreadBase::detach()
{
return pthread_detach(tid);
}
/****************************************************************
@功能:连接线程,等待指定的线程结束,并回收其资源
@参数:无
@返回值: 成功:0,失败:-1
******************************************************************/
int ThreadBase::join(pthread_t t)
{
return pthread_join(t, NULL);
}
/****************************************************************
@功能:线程退出
@参数:无
@返回值: 成功:0,失败:-1
******************************************************************/
int ThreadBase::exit()
{
int ret = 0;
pthread_exit(NULL);
return ret;
}
/****************************************************************
@功能:取消线程
@参数:线程号
@返回值: 成功:0,失败:-1
******************************************************************/
int ThreadBase::cancel(pthread_t t)
{
return pthread_cancel(t);
}
/****************************************************************
@功能:销毁线程
@参数:线程号
@返回值: 成功:0,失败:-1
******************************************************************/
int ThreadBase::destroy()
{
return cancel(tid);
}
/****************************************************************
@功能:线程清理函数
@参数:线程句柄
@返回值: 无
******************************************************************/
void ThreadBase::cleaner(void *pHandle)
{
ThreadBase *p = (ThreadBase *)pHandle;
delete p;
p = NULL;
printf("after clean\n");
}
/****************************************************************
@功能:线程回调函数
@参数:线程句柄
@返回值: 无
******************************************************************/
void *ThreadBase::work(void *pHandle)
{
ThreadBase* pThread = (ThreadBase *)pHandle;
//注册线程处理函数
pthread_cleanup_push(cleaner, pHandle);
pThread->routine(); //线程运行函数
//线程资源释放,参数为非0
pthread_cleanup_pop(1);
return NULL;
}
ThreadDerive.h内容如下:
#ifndef _THREADDERIVE_H_
#define _THREADDERIVE_H_
#include "ThreadBase.h"
//派生类ThreadDerive,公有继承于ThreadBase
class ThreadDerive: public ThreadBase
{
public:
ThreadDerive();
~ThreadDerive();
void init(const int n, const char *str);
void routine();
private:
char buf[512];
int num;
};
#endif
ThreadDerive.cpp内容如下:
#include "ThreadDerive.h"
#include <string.h>
#include <stdio.h>
#include <unistd.h>
//构造函数
ThreadDerive::ThreadDerive()
{
num = 0;
memset(buf, 0, sizeof(buf));
}
//析构函数
ThreadDerive::~ThreadDerive()
{
printf("~ThreadDerive\n");
}
//初始化数据
void ThreadDerive::init(const int n, const char *str)
{
num = n;
strcpy(buf, str);
}
//线程运行函数
void ThreadDerive::routine()
{
printf("I am running\n");
for(int i = 0; i < num; i++)
{
printf("buf = %s, num = %d\n", buf, num);
sleep(1);
}
}
main.cpp内容如下:
#include "ThreadDerive.h"
#include <unistd.h>
#include <stdio.h>
#include <pthread.h>
int main()
{
//一定要动态分配空间,因为基类清理时使用了detele
//不需要人为detele,线程基类已经做处理
ThreadDerive *derive = new ThreadDerive;
derive->init(5, "i am mike");
derive->start();
//usleep(1000*100); //保证线程已经执行
pthread_t tid = derive->self();
printf("tid ========= %lu\n", tid);
derive->detach(); //分离线程
while(1)
{
printf("in the main fun\n");
sleep(1);
}
return 0;
}
编译运行结果如下:
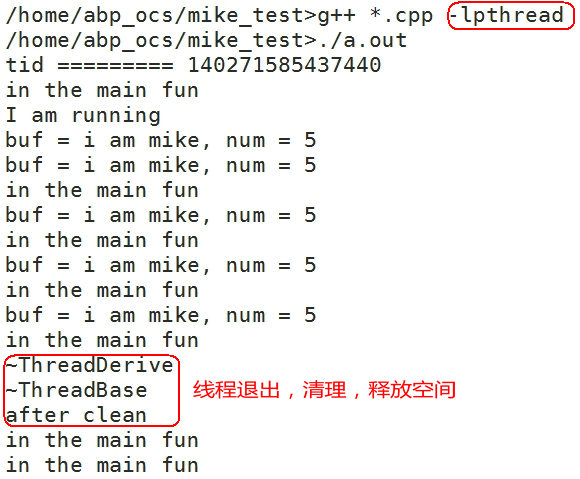